How does react-interpolate work?
VERBto insert (words) into a text or into a conversation.
The way react-interpolate works is by converting a template string like
<h1>Hello {name}. Here is your <b>{order}</b> for {price}.</h1>
to this
<h1>Hello Steve. Here is your <b>iPhone</b> for $799.</h1>
This is useful for cases where we need to show a static template to a dynamic set of users. Example: order details, gift coupons, emailers, etc.
Technical implementation
Defining the regex
First we need to define the regex for how our placeholders will look like.
To begin with, let's define a placeholder as any text that starts with { and ends with }.
The regex for which would look something like this:
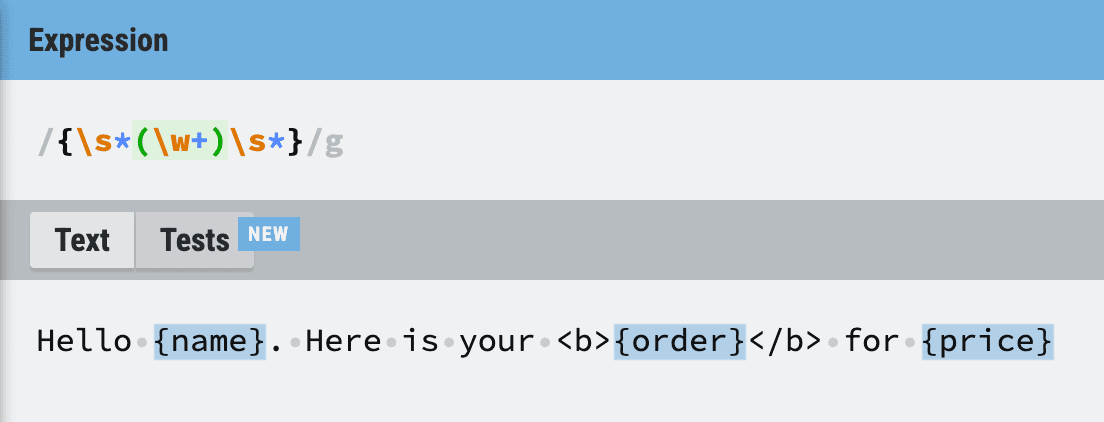
Here is the regexr link to play with: https://regexr.com/7prnk
Parsing the tokens
Once we have the regex, we will parse the given string into tokens.
In this case, these tokens can either be a TEXT or a PLACEHOLDER.
The parser uses a lexer to turn the string into these 2 types of tokens.
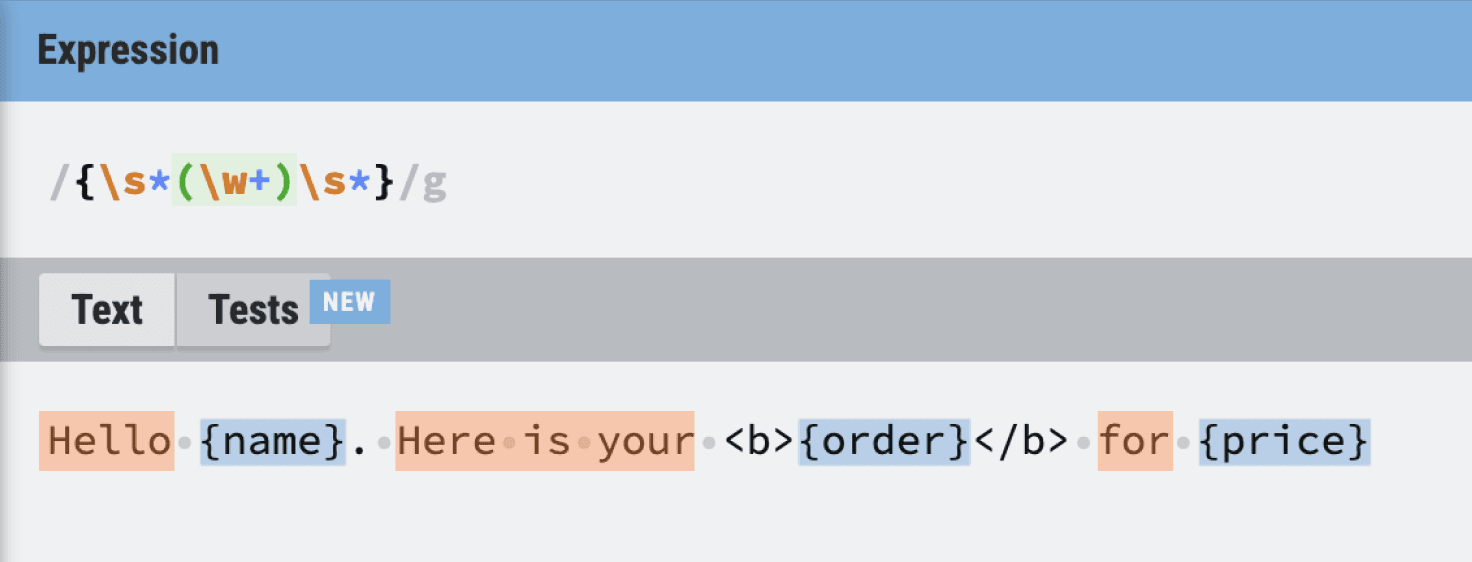
DOM structure
Once we have these tokens, we use a recursive descent parser to create a syntax tree which would resemble the DOM structure. So our template
<h1>Hello {name}. Here is your <b>{order}</b> for {price}</h1>
would result in the following syntax tree with the corresponding tokens 🟦🟧.
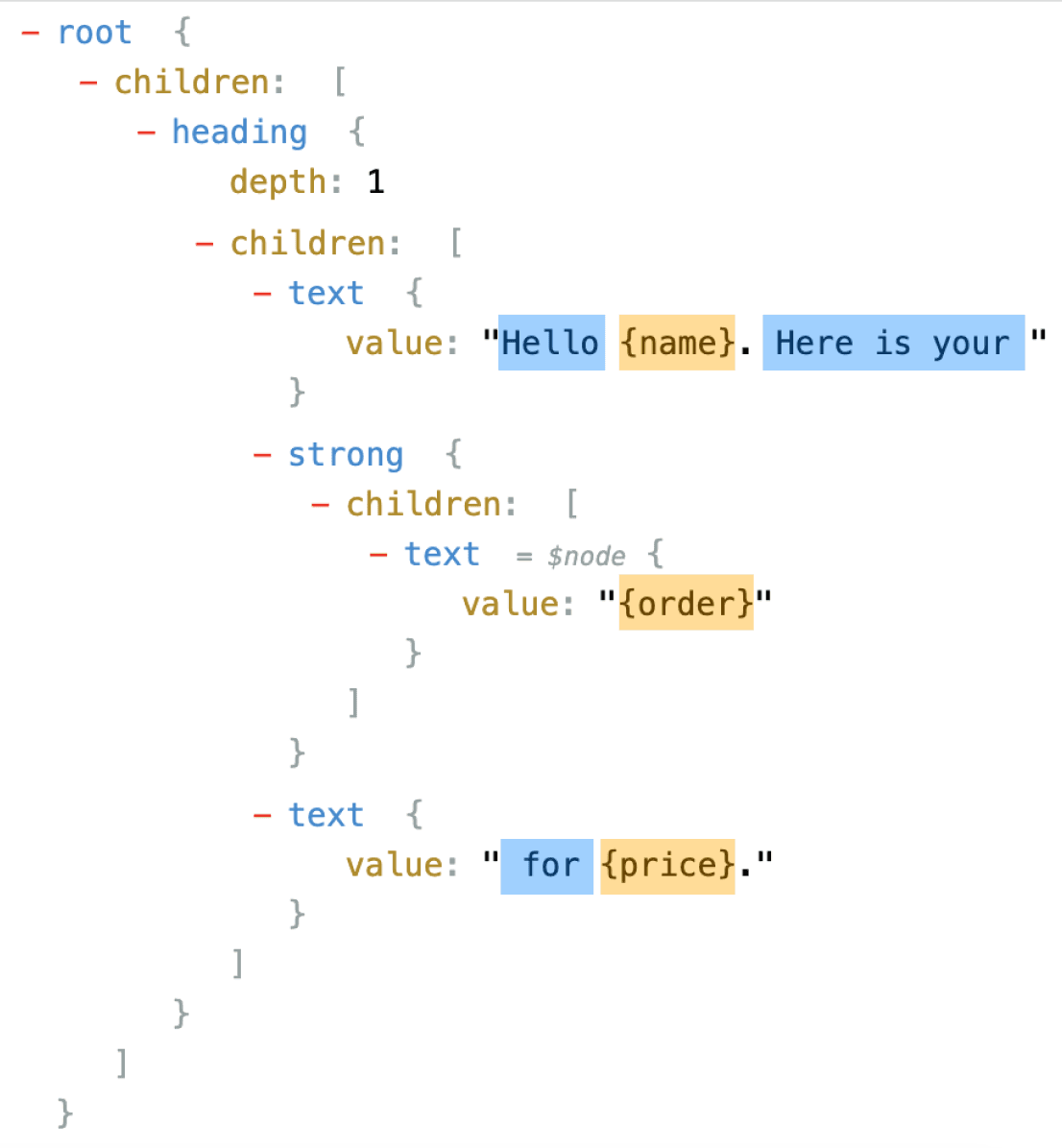
Here is the syntax tree visualiser to explore more: https://astexplorer.net
Value seeder
What's missing? We still need real values to populate our placeholders.
No problem. It is just a plain key-value pair like this:
No problem. It is just a plain key-value pair like this:
{name: "Steve",order: "iPhone",price: "$799"}
We run through our DOM structure and replace the placeholders with the value provided.
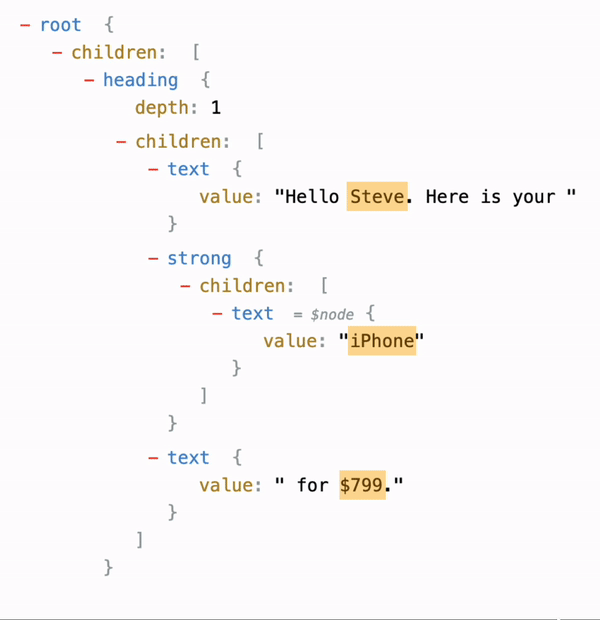
Converting to HTML
On to the final step. How do we convert this DOM like structure to actual HTML?
Remember how React converts JSX → HTML using createElement?
Babel transpiles the JSX into React.createElement function call, that the browser understands. It has the following syntax:
Remember how React converts JSX → HTML using createElement?
Babel transpiles the JSX into React.createElement function call, that the browser understands. It has the following syntax:
React.createElement('h1',null,'Hello Steve. Here is your',React.createElement('b', null, 'iPhone'),'for $799');
This is similar to the syntax tree we have generated above. When we pass our syntax tree to createElement, it would convert it to proper HTML structure and return ✨
<h1>Hello Steve. Here is your <b>iPhone</b> for $799.</h1>
Learning
My initial assumption was this would be basic find-and-replace using regex.But I'm very impressed to learn about other things in the process.
parser → tokenisation → abstract syntax tree → createElement → DOM structure